# 数组复合
IDL 数组复合类型指结构类型数组、枚举类型数组、别名数组、序列类型数组。例如:
//IDL
struct UserDataType{
//序列类型数组
sequence<short> seqs[2];
//别名数组
myString str[3];
//枚举类型数组
Color color[2];
//聚合体数组
U u[2];
//结构体数组
S d[2];
};
1
2
3
4
5
6
7
8
9
10
11
12
13
2
3
4
5
6
7
8
9
10
11
12
13
映射为:
//C++
struct UserDataType {
UserDataType();
UserDataType(const UserDataType &IDL_s);
~UserDataType();
UserDataType& operator= (const UserDataType &IDL_s);
sequence<short> seqs[2];
myString str[3];
Color color[2];
U u[2];
S d[2];
};
1
2
3
4
5
6
7
8
9
10
11
12
13
2
3
4
5
6
7
8
9
10
11
12
13
# IDL 定义示例
接口定义语言(IDL)字符串类型在 idl 文档中定义(以结构体为例):
typedef string myString;
enum Color { blue,green};
struct S{
long l;
string str;
};
union U switch(long){
case 1: short s;
default: long l;
};
struct UserDataType{
sequence<short> seqs[2];
myString str[3];
Color color[2];
U u[2];
S d[2];
};
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
# 代码示例
生成测试用例后在 pub.cpp 中创建数据样本后添加赋值语句:
/* 6. 创建一个数据样本 */
/* 建议:该数据需要申请空间后使用,使用后用户需要调用delete_data进行内存等资源的释放*/
UserDataType *instance = NULL;
instance = UserDataTypeTypeSupport::create_data();
instance->seqs[0].ensure_length(2, 2);
instance->seqs[1].ensure_length(2, 2);
instance->seqs[0][0] = 0;
instance->seqs[0][1] = 1;
instance->seqs[1][0] = 2;
instance->seqs[1][1] = 3;
char str0[10] = "myString0";
char str1[10] = "myString1";
char str2[10] = "myString2";
strcpy(instance->str[0],str0);
strcpy(instance->str[1], str1);
strcpy(instance->str[2], str2);
instance->color[0] = Color::green;
instance->color[1] = Color::blue;
instance->u[0].__d = 1;
instance->u[0].s(10);
instance->u[1].__d = 0;
instance->u[1].l(100);
char dstr0[10] = "dString0";
char dstr1[10] = "dString1";
instance->d[0].l = 11;
strcpy(instance->d[0].str, str0);
instance->d[1].l = 12;
strcpy(instance->d[1].str, str1);
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
# 数据收发示例:
图 1 为使用 IDL 定义示例 中结构体生成的测试 demo,在 Windows 系统上启动发布端、在 Linux 系统启动订阅端后数据收发情况。
图中标号 1 为订阅端接收到发布端一次完整数据的情况显示。
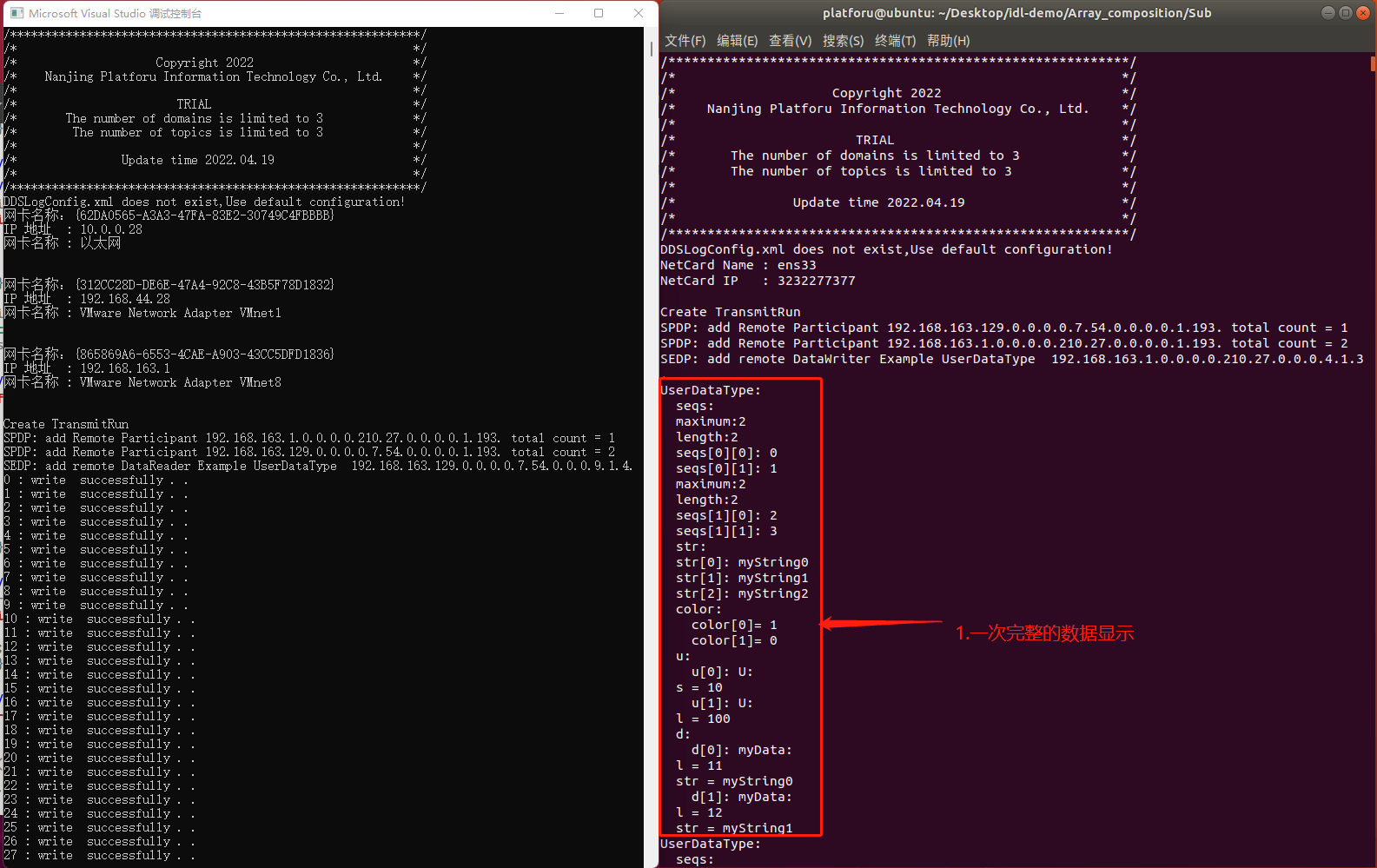
图 1 数组复合类型数据收发示例(Windows->Linux)
图中标号 1 为订阅端接收到发布端一次完整数据的情况显示。
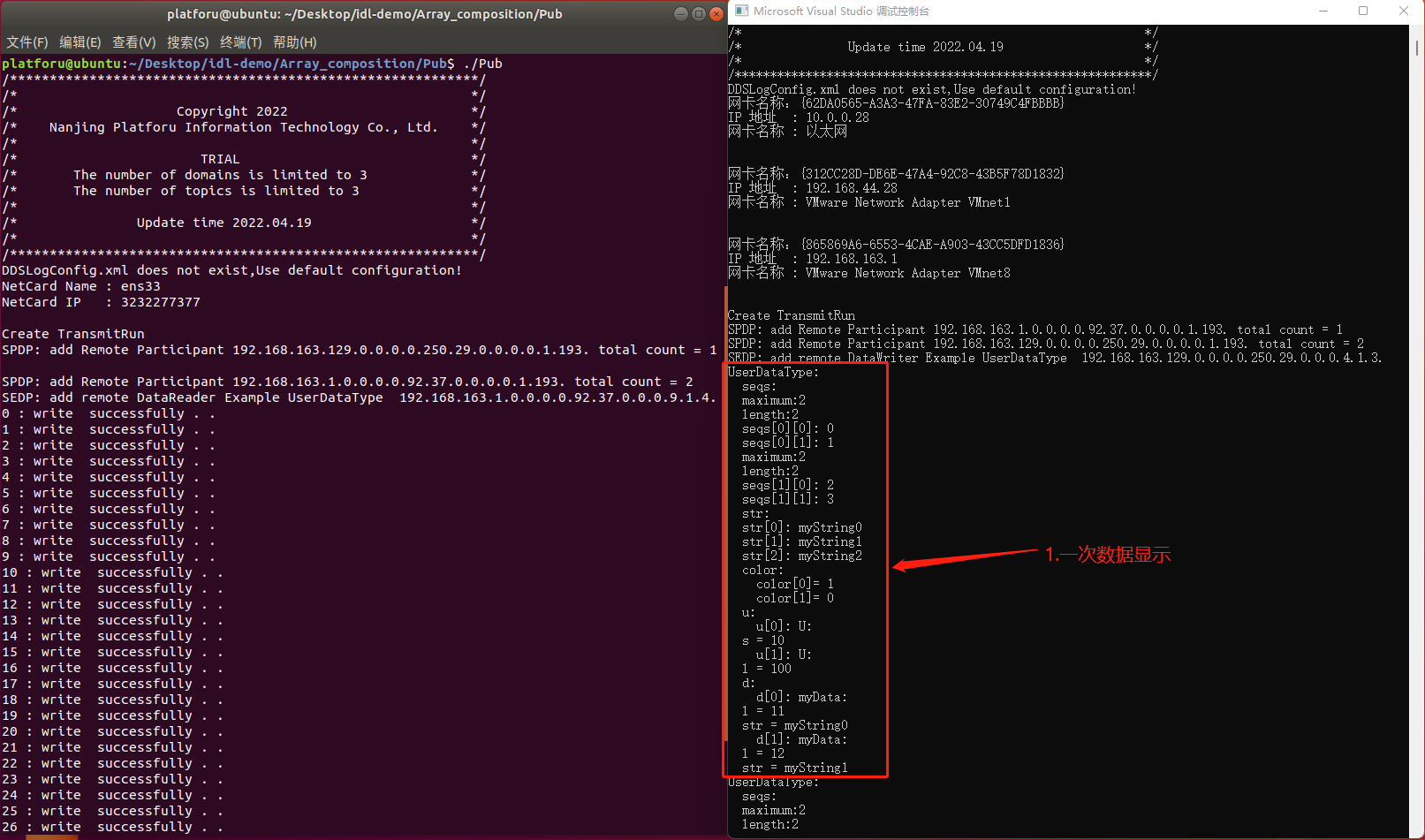
图 2 数组复合类型数据收发示例(Linux -> Windows)
← 取别名(typedef) 序列复合 →